Laravel 11: Streamlining PHP Development with Bold Architectural Shifts
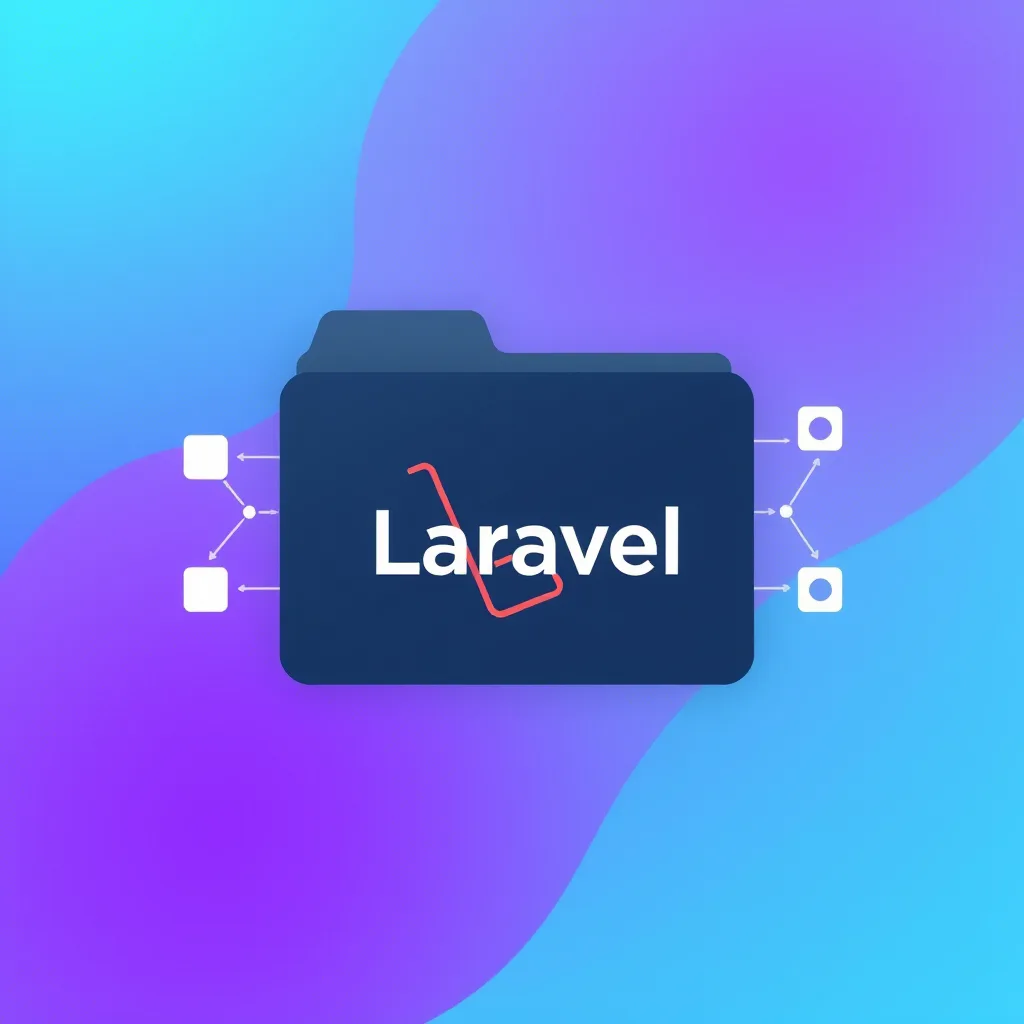
Laravel 11: Embracing Simplicity and Power in Modern Web Development
The Laravel framework has long been celebrated for its elegant syntax, developer-centric design, and vibrant ecosystem. With each iteration, Laravel redefines what it means to deliver a seamless development experience. The release of Laravel 11 marks a pivotal moment in the framework’s evolution, introducing bold architectural changes that prioritize simplicity, performance, and adaptability. By streamlining directory structures, eliminating legacy components, and reimagining data handling, Laravel 11 sets a new standard for modern PHP frameworks. Let’s dive into these transformations and explore how they empower developers to build better applications, faster.
A Leaner Core: Farewell to HTTP Kernel, Console Kernel, and Attribute Casting
1. Simplifying Middleware and Commands: The Removal of Kernels
Historically, the App\Http\Kernel
and App\Console\Kernel
classes were cornerstones of Laravel’s request and command lifecycle management. The HTTP Kernel centralized middleware configuration, while the Console Kernel handled task scheduling. In Laravel 11, these classes have been removed—a move that underscores the framework’s shift toward “convention over configuration.”
What Changed?
- Middleware Registration: Now handled directly in
bootstrap/app.php
or service providers.
// bootstrap/app.php
->withMiddleware(function (Middleware $middleware) {
$middleware->web(append: [Authenticate::class]);
})
- Command Scheduling: Migrated to
AppServiceProvider
:
// AppServiceProvider.php
public function boot(): void {
$this->callAfterResolving(Schedule::class, function (Schedule $schedule) {
$schedule->command('emails:send')->daily();
});
}
Why It Matters
By eliminating explicit Kernel classes, Laravel reduces boilerplate and embraces intuitive defaults. Newcomers face fewer entry barriers, while veterans gain flexibility to override conventions when needed. The result? A cleaner codebase that’s easier to navigate and maintain.
2. Attribute Casting: A Shift Toward Explicit Data Handling
Laravel’s built-in attribute casting, which automatically converted Eloquent model attributes to specified data types, has been deprecated. Instead, developers are encouraged to use accessors and mutators for explicit data transformation.
Before vs. After
// Laravel 10: Implicit casting
protected $casts = ['is_active' => 'boolean'];
// Laravel 11: Explicit accessors/mutators
public function getIsActiveAttribute($value): bool {
return (bool) $value;
}
public function setIsActiveAttribute($value): void {
$this->attributes['is_active'] = (bool) $value;
}
Trade-offs and Benefits
- Pros: Greater transparency, reduced “magic,” and finer control over data flow.
- Cons: Slightly more boilerplate. However, the clarity gained outweighs the extra effort, especially in complex applications.
This change aligns with Laravel’s philosophy of empowering developers through intentional, readable code—a win for long-term maintainability.
Directory Structure Overhaul: Less Clutter, More Clarity
Laravel 11 introduces a flatter, more intuitive project layout designed to reduce cognitive overhead:
Key Changes
- Merged Directories: The
app/Http
andapp/Console
folders are consolidated into a unifiedapp
directory, grouping controllers, middleware, and commands by feature or domain. - Bootstrap Consolidation: The
bootstrap/app.php
file now serves as the central hub for service configuration. - Modular Focus: Developers can organize code into domain-specific modules (e.g.,
app/Users
for user-related logic), promoting scalable architecture.
Example Structure
app/
├─ Models/
├─ Services/
├─ Users/
│ ├─ Controllers/
│ ├─ Requests/
│ └─ Policies/
└─ Providers/
This reorganization reflects Laravel’s commitment to domain-driven design (DDD) and modern application patterns.
Community Response: Praise, Pushback, and Progress
The Laravel community has met these changes with enthusiasm—and a few questions.
Positive Feedback
- Developers applaud the reduced boilerplate and simplified structure, calling it “a breath of fresh air.”
- The shift toward explicit coding has been praised for minimizing hidden behaviors.
Concerns and Adaptations
- Some long-time users expressed nostalgia for attribute casting’s convenience.
- Migration guides and community packages (e.g., reintroducing casting via traits) have emerged to ease transitions.
As with any evolution, adaptation takes time—but Laravel’s robust documentation and active forums provide ample support.
Looking Ahead: Laravel 12 and the Future
Laravel 11 sets the stage for even more ambitious innovations. Early discussions about Laravel 12 hint at:
- Optional Kernel Scaffolding: Balancing backward compatibility with modern defaults.
- Enhanced Tooling: Improved
artisan
commands for scaffolding modular code. - Performance Optimizations: Faster routing and service container resolution.
The framework’s trajectory is clear: double down on developer productivity while staying true to its elegant roots.
Conclusion: Why Laravel 11 Matters
Laravel 11 isn’t just an update—it’s a manifesto. By stripping away redundancy and championing explicit, purposeful code, the framework empowers developers to build scalable, maintainable applications without compromise. Whether you’re a seasoned Laravel developer or a newcomer, these changes invite you to rethink conventions, embrace simplicity, and join a community that’s shaping the future of PHP.
Further Reading
- Laravel 11 Official Release Notes
- Laracasts: Navigating Laravel 11
- Community Packages for Attribute Casting
Let us know your thoughts on Laravel 11’s changes in the comments below! 💬